// [Object Info] //////////////////////////////////////////////////////////////
Now we'll look at layout objects, button clicks and handling multiple
buttons.
We'll make a small panel that will give us information about a selected
object when we click on the header. We'll make a function called 'drawpanel'
that can be called at any time to initialise or update the entire panel.
We'll set some variables to control our panel, and then draw our panel.
We'll also make a variable to store our target object, so that our panel
doesn't need the object to be passed to it.
We'll need to hook the BTC object to detect button clicks. Here we only have
one to check, and if we find it we'll send a TTC packet that requests the
current layout editor selection.
We'll hook the AXM packet to watch for replies, and discard any that are not
of the correct type. We'll find the first object and store it before calling
for the panel to be redrawn.
Finally, we'll define our function to draw the panel. We'll make the first
button clickable, and then, if there is an object saved, we'll loop through
the fields and output them as a row. Note that we're increasing the id value
as we go - we'll use this next to delete any leftover buttons from a previous
draw using a BFN packet.
objectinfo.php:
command line:
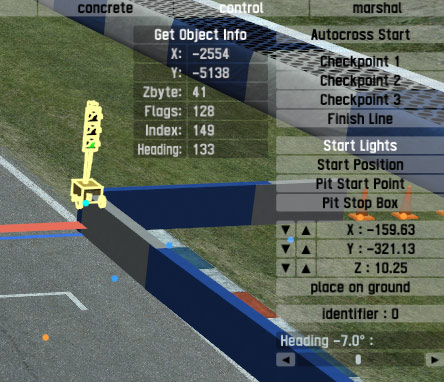
Now we'll look at layout objects, button clicks and handling multiple
buttons.
We'll make a small panel that will give us information about a selected
object when we click on the header. We'll make a function called 'drawpanel'
that can be called at any time to initialise or update the entire panel.
We'll set some variables to control our panel, and then draw our panel.
We'll also make a variable to store our target object, so that our panel
doesn't need the object to be passed to it.
We'll need to hook the BTC object to detect button clicks. Here we only have
one to check, and if we find it we'll send a TTC packet that requests the
current layout editor selection.
We'll hook the AXM packet to watch for replies, and discard any that are not
of the correct type. We'll find the first object and store it before calling
for the panel to be redrawn.
Finally, we'll define our function to draw the panel. We'll make the first
button clickable, and then, if there is an object saved, we'll loop through
the fields and output them as a row. Note that we're increasing the id value
as we go - we'll use this next to delete any leftover buttons from a previous
draw using a BFN packet.
objectinfo.php:
<?php
function PIE_settings()
{
return array('flags' => ISF_LOCAL,
'insimname' => 'ObjectInfo');
}
function PIE_start()
{
$PIE = PIE::connect();
$PIE->set('panelbaseid', 20);
$PIE->set('paneltop', 7);
$PIE->set('panelleft', 154);
$PIE->set('panelwidth', 20);
$PIE->set('panelbheight', 5);
$PIE->set('object', array());
drawpanel();
}
function PIE_BTC($data)
{
$PIE = PIE::connect();
$bid = $PIE->get('panelbaseid');
if ($data['ClickID'] == $bid)
PIE_sendTTC(TTC_SEL);
}
function PIE_AXM($data)
{
if ($data['PMOAction'] != PMO_TTC_SEL)
return;
if ($data['NumO'] < 1)
$object = array();
else
$object = $data['Info'][0];
$PIE = PIE::connect();
$PIE->set('object', $object);
drawpanel();
}
function drawpanel()
{
$PIE = PIE::connect();
$bid = $PIE->get('panelbaseid');
$top = $PIE->get('paneltop');
$left = $PIE->get('panelleft');
$width = $PIE->get('panelwidth');
$bheight = $PIE->get('panelbheight');
$object = $PIE->get('object');
$id = $bid;
$packet = array('Type' => ISP_BTN,
'ClickID' => $id++,
'BStyle' => ISB_DARK | ISB_CLICK,
'T' => $top,
'L' => $left,
'W' => $width,
'H' => $bheight,
'Text' => '^7Get Object Info');
PIE_sendpacket($packet);
$row = 1;
foreach($object as $k=>$v)
{
$packet = array('Type' => ISP_BTN,
'ClickID' => $id++,
'BStyle' => ISB_DARK | ISB_RIGHT,
'T' => $top + ($row * $bheight),
'L' => $left,
'W' => 8,
'H' => $bheight,
'Text' => $k.':');
PIE_sendpacket($packet);
$packet = array('Type' => ISP_BTN,
'ClickID' => $id++,
'BStyle' => ISB_DARK | ISB_LEFT,
'T' => $top + ($row * $bheight),
'L' => $left+8,
'W' => 12,
'H' => $bheight,
'Text' => $v);
PIE_sendpacket($packet);
$row += 1;
}
$max = $bid + 12;
if ($id < $max)
PIE_sendpacket(array( 'Type' => ISP_BFN,
'SubType' => BFN_DEL_BTN,
'ClickID' => $id,
'ClickMax' => $max));
}
?>
> pie tutorials\objectinfo.php