Buttons are the first thing anyone should learn about if they are new to editing the default LFSLapper ('lapper') code, or want to add their own code.
Buttons are name given by LFS to any text (whether on clear or grey coloured background) or just coloured backgrounds that appear on the screen. Every InSim, including lapper, uses same convention. LFS InSim allows a maximum of 240 buttons on screen at any time. LFS can display normal buttons in these four screens:
- main entry screen
- race setup screen
- in game
- SHIFT+U mode
Usually the first, and main thing people want to change in lapper is the 'Welcome' message when you connect to your server.
Buttons are the easiest thing to do in lapper. If you can't edit to change them, make your own, or are not willing to experiment, then my advice is to live with whatever the default code is, and tag on any additional add-ons that take your fancy, assuming you know how to add an add-on.
The first part of the standard LFSLapper.lpr file (before any code) tells you about buttons; difference between private and global, what the numbers mean regarding button size and placement, the time setting, the background colour, the text colours.
If it still makes little or no sense, then look at the Welcome message that appears on your screen when you join your server and compare that to the actual code. This Welcome message appears in the OnConnect Event.

The actual text will be in the language section at the bottom of the lapper file, where the code for a particular button will be converted to a text string, eg
main_welc1 = "^7Welcome {0} ^7to ^1LFSLapper ^7powered server !%nl%^2Type ^7!help ^2after leaving garage to see commands.";
where numbers within curly brackets (ie {0}) refer to the variable at the end of code line (in this case its the users Nickname)
and %nl% means text after this goes on new line
and the caret (^) mark with numbers in front of text define the message text colours (e.g. ^7 = white. No number or ^8 both mean light grey)
The -1 in the button code means the button will stay on screen for ever, unless a sub-routine comes up later to close that particular button.
In most cases, you will want to make private buttons, as they are used for the individual driver.
openPrivButton - used when you want individual driver to see on-screen buttons. For example, you don't want the 'Welcome' message to be Global, as everytime someone joined the server, every person already on the server will see same message.
Example of using private buttons -
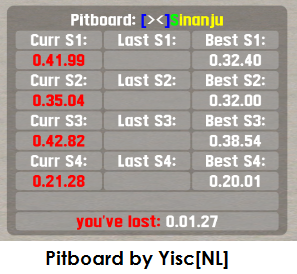
openGlobalButton - used when you want everyone to see same on-screen buttons, such as an admin message.
Another way of looking at the difference is that when racing in LFS, your lap numbers and times will be private (as they only refer to laps and times you've driven), but the results table that appears at end, is Global, as every racer sees this same table.
Sometimes at the end of the line of button code, after the text, there's nothing. Sometimes you will get a single variable, or multiple variables, and others a sub-routine.
Examples;
Nothing
Single Var
Multiple Vars
Sub-routine
A variable will start with a $. As in, $NickName
Variables are placed by using the numbers in curly brackets (as explained above), always starting with zero (0). More than one variable, then use next number {1} ...
In the Welcome message, the button called "pos" uses multiple variables.
Variables are called vars, as in PlayerVars.
The sub-routine may just be a word, or series of words without spaces, such as OnConnectClose.
A Sub-routine in lapper is called a sub.
In most cases, where there's a sub, it means that the button on screen needs pressed for it to do something. For more advanced users, you can use %cpt% to act as a timer, so that when it runs out, the sub in your button is activated.
In most cases, when you make a button, it's only displaying a message, and requires no interaction.
This 'button' may have a clear background, or a light grey background, or a darker grey background. It may just be a background with no text (in code written as ""), or it may have coloured text, which has been centred, justified left, or justified right.
For really experience users, it is possible to make coloured buttons, and even circular buttons. These are really letters and symbols blown up to huge scale.
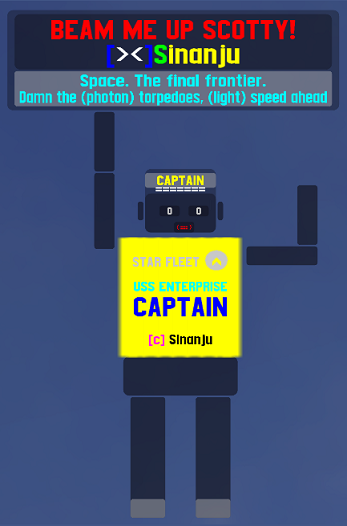
Above image consists of 36 buttons; some with text, some without. In some bits, one button is overlaid onto another to make something appear even darker.
As you can see, buttons don't just have to give you messages!
You can get countdown buttons (explained near top of this post, using %cpt%, and you can even get buttons that change the text colour to simulate blinking, using %at% - look in the 'changes.txt' file included in the /doc directory of lapper on how to use.
Sometimes when you're reading someone's add-on code, you will see that some of the numbers you would expect to see within the button line of text has been changed to strange wording, or wording plus a number, as in
$RTorigL+1
This just means the author has made a GlobalVar, in this case called $RTorigL, which can stand for any number (up to 200) and his button is to be further left, by 1, on what he has specified. If his GlobalVar was 10, then this button would be placed at 11.
Buttons are placed in a 200 x 200 area of your screen. This area is defined by InSim, not lapper.
Extract from InSim 7 documentation regarding placement;
First number is the X heading (left to right), next number is -Y heading (you start at top of screen, and larger numbers place things further down. Next number is width of button. This number, plus X heading shouldn't add to more than 200, or your button will be placed partly off screen. Next number is height of button or text. Again, this number, plus -Y heading shouldn't exceed 200 or will disappear off bottom of screen.
Occasionally you will see in both the main LFSLapper.lpr and some people's add-on lapper scripts, that the last number (after the -1 in above examples) is not shown as a number, but as ISB_ text.
Some of these numbers/text are interchangeable.
- 16 or ISB_LIGHT for light button
- 32 or ISB_DARK for dark button
- 64 or ISB_LEFT to align text to left
- 128 or ISB_RIGHT to align text to right
GlobalVars used for placing buttons are great if you're developing a script. If you define your var and all your buttons use this var, then buttons will be placed where you wanted. If you're not happy where all these buttons land up, you just change the single GlobalVar - NO need to change relevant detail on every single button.
Most buttons that require interaction (user to click), usually have the time set to -1. When clicked, the first thing that usually needs to happen is you have to close the button that was clicked (closePrivButton / closeGlobalButton), so you will need to learn about these too. When you get more experienced, likely you'll want to start using 'closeButtonRegex' too.
When making buttons, you will tend to set a unique id for each button, such as 'welc' or 'clos' (see OnConnect section).
However, it is possible to re-use these names (might be slightly confusing if you do) - usually best if they've already closed, else they will be re-written but with different text messages.
In some of my code scripts, I do this deliberately. One of my buttons is called "hud_message". If something happens, a message appears on the hud (long button above mirror!). If something else happens, this new button with same name, but different message, will overwrite the first hud message.
Some of this may likely seem complicated. Some of it is, but I've learned how to do it because I've experimented a lot on making simple buttons, gradually getting onto more advanced buttons and advanced way of using buttons.
Note that buttons are not the only way to put just text on screen. Look at the lapper script for examples of privMsg and globalMsg. These are output at top left of screen, where you normally see messages when people write them on a server. LFS itself outputs these as global messages, as everyone on server see's them.
The buttons described above (openPrivButton, openGlobalButton, privMsg, globalMsg) is not a definitive list. There are other buttons too, including;
openPrivTextButton
privRCM
GlobalRcm
Look in the standard lapper file to see examples of how they are used.
Also, for these buttons, and most other things, read the changes.txt file in the /docs folder.
If you are going to experiment, best to do so with different copy of lapper to what you may have on your server, and this experimental copy of lapper connecting to your own pc via Multiplayer/start new host/local (visibility). Remember you need to type insim/29999 when you connect to LFS, and the password you use MUST match the password in the default_1.ini file (which can be edited in NotePad).
If you've managed to read this whole post, likely means you have the stamina to learn how to code your own buttons!
Good luck!
Buttons are name given by LFS to any text (whether on clear or grey coloured background) or just coloured backgrounds that appear on the screen. Every InSim, including lapper, uses same convention. LFS InSim allows a maximum of 240 buttons on screen at any time. LFS can display normal buttons in these four screens:
- main entry screen
- race setup screen
- in game
- SHIFT+U mode
Usually the first, and main thing people want to change in lapper is the 'Welcome' message when you connect to your server.
Buttons are the easiest thing to do in lapper. If you can't edit to change them, make your own, or are not willing to experiment, then my advice is to live with whatever the default code is, and tag on any additional add-ons that take your fancy, assuming you know how to add an add-on.
The first part of the standard LFSLapper.lpr file (before any code) tells you about buttons; difference between private and global, what the numbers mean regarding button size and placement, the time setting, the background colour, the text colours.
If it still makes little or no sense, then look at the Welcome message that appears on your screen when you join your server and compare that to the actual code. This Welcome message appears in the OnConnect Event.
The actual text will be in the language section at the bottom of the lapper file, where the code for a particular button will be converted to a text string, eg
main_welc1 = "^7Welcome {0} ^7to ^1LFSLapper ^7powered server !%nl%^2Type ^7!help ^2after leaving garage to see commands.";
where numbers within curly brackets (ie {0}) refer to the variable at the end of code line (in this case its the users Nickname)
and %nl% means text after this goes on new line
and the caret (^) mark with numbers in front of text define the message text colours (e.g. ^7 = white. No number or ^8 both mean light grey)
The -1 in the button code means the button will stay on screen for ever, unless a sub-routine comes up later to close that particular button.
In most cases, you will want to make private buttons, as they are used for the individual driver.
openPrivButton - used when you want individual driver to see on-screen buttons. For example, you don't want the 'Welcome' message to be Global, as everytime someone joined the server, every person already on the server will see same message.
Example of using private buttons -
openGlobalButton - used when you want everyone to see same on-screen buttons, such as an admin message.
Another way of looking at the difference is that when racing in LFS, your lap numbers and times will be private (as they only refer to laps and times you've driven), but the results table that appears at end, is Global, as every racer sees this same table.
Sometimes at the end of the line of button code, after the text, there's nothing. Sometimes you will get a single variable, or multiple variables, and others a sub-routine.
Examples;
Nothing
openPrivButton( "blank",25,50,150,60,12,-1,32,"");
Single Var
openPrivButton( "welc",25,50,150,15,12,-1,0, langEngine("%{main_welc1}%", $NickName ) );
Multiple Vars
openPrivButton( "pos",25,80,150,10,8,-1,0,langEngine("%{main_welc2}%",$Posabs,$Posqual,$Groupqual));
Sub-routine
openPrivButton( "clos",78,120,20,10,10,-1,32,langEngine("%{main_accept}%"),OnConnectClose );
A variable will start with a $. As in, $NickName
Variables are placed by using the numbers in curly brackets (as explained above), always starting with zero (0). More than one variable, then use next number {1} ...
In the Welcome message, the button called "pos" uses multiple variables.
Variables are called vars, as in PlayerVars.
The sub-routine may just be a word, or series of words without spaces, such as OnConnectClose.
A Sub-routine in lapper is called a sub.
In most cases, where there's a sub, it means that the button on screen needs pressed for it to do something. For more advanced users, you can use %cpt% to act as a timer, so that when it runs out, the sub in your button is activated.
In most cases, when you make a button, it's only displaying a message, and requires no interaction.
This 'button' may have a clear background, or a light grey background, or a darker grey background. It may just be a background with no text (in code written as ""), or it may have coloured text, which has been centred, justified left, or justified right.
For really experience users, it is possible to make coloured buttons, and even circular buttons. These are really letters and symbols blown up to huge scale.
Above image consists of 36 buttons; some with text, some without. In some bits, one button is overlaid onto another to make something appear even darker.
As you can see, buttons don't just have to give you messages!
You can get countdown buttons (explained near top of this post, using %cpt%, and you can even get buttons that change the text colour to simulate blinking, using %at% - look in the 'changes.txt' file included in the /doc directory of lapper on how to use.
Sometimes when you're reading someone's add-on code, you will see that some of the numbers you would expect to see within the button line of text has been changed to strange wording, or wording plus a number, as in
$RTorigL+1
This just means the author has made a GlobalVar, in this case called $RTorigL, which can stand for any number (up to 200) and his button is to be further left, by 1, on what he has specified. If his GlobalVar was 10, then this button would be placed at 11.
Buttons are placed in a 200 x 200 area of your screen. This area is defined by InSim, not lapper.
Extract from InSim 7 documentation regarding placement;
First number is the X heading (left to right), next number is -Y heading (you start at top of screen, and larger numbers place things further down. Next number is width of button. This number, plus X heading shouldn't add to more than 200, or your button will be placed partly off screen. Next number is height of button or text. Again, this number, plus -Y heading shouldn't exceed 200 or will disappear off bottom of screen.
Occasionally you will see in both the main LFSLapper.lpr and some people's add-on lapper scripts, that the last number (after the -1 in above examples) is not shown as a number, but as ISB_ text.
Some of these numbers/text are interchangeable.
- 16 or ISB_LIGHT for light button
- 32 or ISB_DARK for dark button
- 64 or ISB_LEFT to align text to left
- 128 or ISB_RIGHT to align text to right
GlobalVars used for placing buttons are great if you're developing a script. If you define your var and all your buttons use this var, then buttons will be placed where you wanted. If you're not happy where all these buttons land up, you just change the single GlobalVar - NO need to change relevant detail on every single button.
Most buttons that require interaction (user to click), usually have the time set to -1. When clicked, the first thing that usually needs to happen is you have to close the button that was clicked (closePrivButton / closeGlobalButton), so you will need to learn about these too. When you get more experienced, likely you'll want to start using 'closeButtonRegex' too.
When making buttons, you will tend to set a unique id for each button, such as 'welc' or 'clos' (see OnConnect section).
However, it is possible to re-use these names (might be slightly confusing if you do) - usually best if they've already closed, else they will be re-written but with different text messages.
In some of my code scripts, I do this deliberately. One of my buttons is called "hud_message". If something happens, a message appears on the hud (long button above mirror!). If something else happens, this new button with same name, but different message, will overwrite the first hud message.
Some of this may likely seem complicated. Some of it is, but I've learned how to do it because I've experimented a lot on making simple buttons, gradually getting onto more advanced buttons and advanced way of using buttons.
Note that buttons are not the only way to put just text on screen. Look at the lapper script for examples of privMsg and globalMsg. These are output at top left of screen, where you normally see messages when people write them on a server. LFS itself outputs these as global messages, as everyone on server see's them.
The buttons described above (openPrivButton, openGlobalButton, privMsg, globalMsg) is not a definitive list. There are other buttons too, including;
openPrivTextButton
privRCM
GlobalRcm
Look in the standard lapper file to see examples of how they are used.
Also, for these buttons, and most other things, read the changes.txt file in the /docs folder.
If you are going to experiment, best to do so with different copy of lapper to what you may have on your server, and this experimental copy of lapper connecting to your own pc via Multiplayer/start new host/local (visibility). Remember you need to type insim/29999 when you connect to LFS, and the password you use MUST match the password in the default_1.ini file (which can be edited in NotePad).
If you've managed to read this whole post, likely means you have the stamina to learn how to code your own buttons!
Good luck!