// [Headsup] //////////////////////////////////////////////////////////////////
Now, let's make ourselves a local headsup speedometer. We'll need to set up
OutGauge in LFS for this, by editing the cfg.txt file (with LFS closed). We'll
need to set 'OutGauge IP' to localhost, choose a free port for 'OutGauge
Port', set 'OutGauge Mode' to 2 (local and viewed players) and 'OutGauge
Delay' to 10 (1/100th second).
In our 'PIE_settings' function, we need to add 'outgaugehost' and
'outgaugeport' settings to match.
We will also define a 'PIE_OutGauge' function, to catch the packets once
they begin arriving. Inside this function, we will format the car's speed and
send it to screen as an InSim button. As this packet uses the UDP protocol, we
will first check the timestamp and discard any packets that have been received
out of order.
The speed supplied in the OutGauge packet is in metres per second, so we
need to multiply by 2.2 to get our speed in miles per hour, or 3.5398 to get
kilomtres per hour.
LFS's cfg.txt file, OutGauge lines only:
headsup.php:
command line:
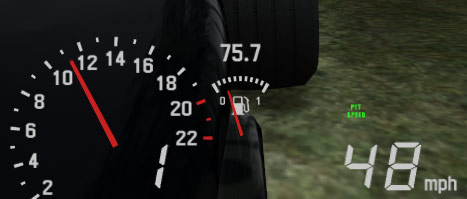
// [Headsup log] //////////////////////////////////////////////////////////////
Finally, let's use some more native PHP. We'll take the previous headsup
program and add some logging to a file - we'll record our speed in a CSV file,
ready for importing into another program.
We'll need to open the file for appending at the start and close it at the
end in order to save overhead - we don't need to do this every time we write
as we can store the file pointer in a PIE variable. Note, we'll need to use
the constant 'PIE_SCRIPTDIR' to reference the folder that the PIE script is
running from, if we want to use relative file paths.
In our PIE_OutGauge function, we simply check the pointer is valid and write
a line to the file. We'll write the time and the speed separated by a comma,
and a PHP constant for the carriage at the end of the line.
headsuplog.php:
command line:
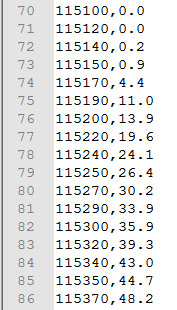
Now, let's make ourselves a local headsup speedometer. We'll need to set up
OutGauge in LFS for this, by editing the cfg.txt file (with LFS closed). We'll
need to set 'OutGauge IP' to localhost, choose a free port for 'OutGauge
Port', set 'OutGauge Mode' to 2 (local and viewed players) and 'OutGauge
Delay' to 10 (1/100th second).
In our 'PIE_settings' function, we need to add 'outgaugehost' and
'outgaugeport' settings to match.
We will also define a 'PIE_OutGauge' function, to catch the packets once
they begin arriving. Inside this function, we will format the car's speed and
send it to screen as an InSim button. As this packet uses the UDP protocol, we
will first check the timestamp and discard any packets that have been received
out of order.
The speed supplied in the OutGauge packet is in metres per second, so we
need to multiply by 2.2 to get our speed in miles per hour, or 3.5398 to get
kilomtres per hour.
LFS's cfg.txt file, OutGauge lines only:
OutGauge Mode 2
OutGauge Delay 10
OutGauge IP 127.0.0.1
OutGauge Port 49999
headsup.php:
<?php
function PIE_settings()
{
return array('flags' => ISF_LOCAL,
'outgaugehost' => '127.0.0.1',
'outgaugeport' => 49999);
}
function PIE_OutGauge($data)
{
$PIE = PIE::connect();
$lasttime = $PIE->get('lastOutGauge');
$thistime = $data['Time'];
$PIE->set('lastOutGauge', $thistime);
if ($lasttime > $thistime)
return;
$speed = number_format(($data['Speed']*3.5398), 1);
PIE_sendpacket(array( 'Type' => ISP_BTN,
'ClickID' => 7,
'BStyle' => 0,
'T' => 147,
'L' => 90,
'W' => 20,
'H' => 8,
'Text' => $speed));
}
?>
> pie tutorials\headsup.php
// [Headsup log] //////////////////////////////////////////////////////////////
Finally, let's use some more native PHP. We'll take the previous headsup
program and add some logging to a file - we'll record our speed in a CSV file,
ready for importing into another program.
We'll need to open the file for appending at the start and close it at the
end in order to save overhead - we don't need to do this every time we write
as we can store the file pointer in a PIE variable. Note, we'll need to use
the constant 'PIE_SCRIPTDIR' to reference the folder that the PIE script is
running from, if we want to use relative file paths.
In our PIE_OutGauge function, we simply check the pointer is valid and write
a line to the file. We'll write the time and the speed separated by a comma,
and a PHP constant for the carriage at the end of the line.
headsuplog.php:
<?php
function PIE_settings()
{
return array('flags' => ISF_LOCAL,
'outgaugehost' => '127.0.0.1',
'outgaugeport' => 49999);
}
function PIE_start()
{
$PIE = PIE::connect();
$fp = fopen(PIE_SCRIPTDIR.'speedlog.csv', 'w');
$PIE->set('filepointer', $fp);
}
function PIE_OutGauge($data)
{
$PIE = PIE::connect();
$lasttime = $PIE->get('lastOutGauge');
$thistime = $data['Time'];
$PIE->set('lastOutGauge', $thistime);
if ($lasttime >= $thistime)
return;
$speed = number_format(($data['Speed']*3.5398), 1);
PIE_sendpacket(array( 'Type' => ISP_BTN,
'ClickID' => 7,
'BStyle' => 0,
'T' => 147,
'L' => 90,
'W' => 20,
'H' => 8,
'Text' => $speed));
$fp = $PIE->get('filepointer');
if ($fp)
fwrite($fp, ''.$thistime.','.$speed.PHP_EOL);
}
function PIE_shutdown()
{
$PIE = PIE::connect();
$fp = $PIE->get('filepointer');
fclose($fp);
}
?>
> pie tutorials\headsuplog.php