// [Random lights] ////////////////////////////////////////////////////////////
Now let's look at another way to repeat actions, how to store and retrieve
values, and, as we're getting more complicated now, let's look at some simple
live debugging too.
We're going to make a program that will assign random lights to startlight
objects at a set rate, and then we'll fiddle with it while it runs.
In the previous examples we have not needed to communicate between
functions, but this time we're going to count how many times the lights have
changed. We will need to keep track of the count between multiple calls of the
light-changing function. To do this we'll use the PIE object - an object that
contains InSim information, helper functions, and is always there in the
background.
Important note: To use the PIE object, we must first connect to it. We do
this by assigning it to the $PIE variable. This variable can then be used
throughout the function. You must do this inside each function that needs to
use the PIE object. It's fatal PHP error to forget this step, and PIE can't
protect you from forgetting to do this part, sorry!
In our 'PIE_start' function, once we have a $PIE variable, we can use the
'set' function to store data for later use. We'll store some id numbers to
specify which lights to change, a delay to regulate the speed, and a counter
just for example.
We've got a new function 'PIE_clock', which is called every second with the
unix timestamp as a parameter. We'll connect to the PIE object, retrieve the
delay and then use that to call the 'randomiselights' function every so many
seconds.
In our 'randomiselights' function, we again connect to the PIE object first,
as we'll need our stored data. We'll retrieve our array of light ids and loop
through them - for each light we'll set a random set of bulbs. We'll use the
OCO packet to control our lights, specifying objects by the identifier set in
LFS.
Finally, we'll add one to our counter.
Note, you will need the some startlight objects with the correct identifier
id on your layout, and the layout must be optimised before InSim can control
them. The easiest way to do this is to restart the currect session.
Once we see the lights changing, we can do some live debugging:
We can inspect our counter with a text command ";piepeeku lightchanges". We
can also use this to see our current delay with ";piepeeku lightdelay", and we
can alter it (let's say we'll set it to 7 instead of 4), with ";piepokeu
lightdelay 7".
Note that these commands are limited to admins. In host mode, PIE uses the
server admin status to determine who's allowed to run these commands. In local
mode, you will need to specify your account name in the settings.
randomlights.php:
command line:
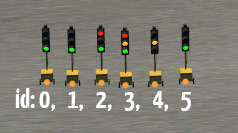
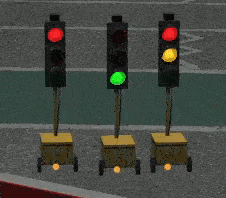
// [Debugging in code] ////////////////////////////////////////////////////////
Now, we'll do some more debugging - you can never have enough ways to find
out what's going on! We'll take our previous lights program and modify the
randomiselights function.
We'll build two strings to tell us what's going on as each light is set, one
for console debugging now and another saved in an array for outputting to chat
later.
We use the 'PIE_debugout' function to optionally output debugging messages
to the PIE console. The first parameter is the level of debugging that should
be required for this message to be output, the second is the message itself.
Alternatively, we can use the 'PIE_debugcheck' function to see if a certain
level of debugging is enabled, in order to deal with the debugging ourselves.
Here we'll send it to chat. We'll use a different debugging level so that we
can toggle these two debugging options independently.
We also have a new helper function 'textbulbs', to more easily see which
bulbs should be set for comparison. (Note, this is correct only for startlight
objects, main lights follow a different pattern.)
randomlightsdebug.php:
command line:
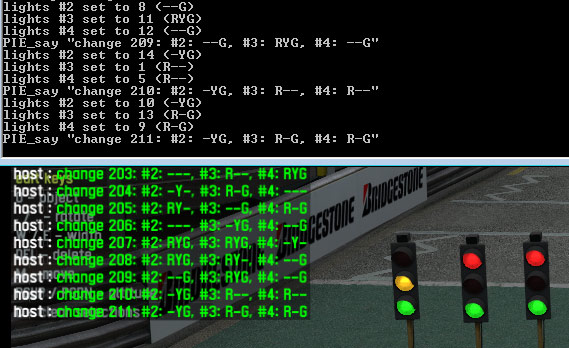
Now let's look at another way to repeat actions, how to store and retrieve
values, and, as we're getting more complicated now, let's look at some simple
live debugging too.
We're going to make a program that will assign random lights to startlight
objects at a set rate, and then we'll fiddle with it while it runs.
In the previous examples we have not needed to communicate between
functions, but this time we're going to count how many times the lights have
changed. We will need to keep track of the count between multiple calls of the
light-changing function. To do this we'll use the PIE object - an object that
contains InSim information, helper functions, and is always there in the
background.
Important note: To use the PIE object, we must first connect to it. We do
this by assigning it to the $PIE variable. This variable can then be used
throughout the function. You must do this inside each function that needs to
use the PIE object. It's fatal PHP error to forget this step, and PIE can't
protect you from forgetting to do this part, sorry!
In our 'PIE_start' function, once we have a $PIE variable, we can use the
'set' function to store data for later use. We'll store some id numbers to
specify which lights to change, a delay to regulate the speed, and a counter
just for example.
We've got a new function 'PIE_clock', which is called every second with the
unix timestamp as a parameter. We'll connect to the PIE object, retrieve the
delay and then use that to call the 'randomiselights' function every so many
seconds.
In our 'randomiselights' function, we again connect to the PIE object first,
as we'll need our stored data. We'll retrieve our array of light ids and loop
through them - for each light we'll set a random set of bulbs. We'll use the
OCO packet to control our lights, specifying objects by the identifier set in
LFS.
Finally, we'll add one to our counter.
Note, you will need the some startlight objects with the correct identifier
id on your layout, and the layout must be optimised before InSim can control
them. The easiest way to do this is to restart the currect session.
Once we see the lights changing, we can do some live debugging:
We can inspect our counter with a text command ";piepeeku lightchanges". We
can also use this to see our current delay with ";piepeeku lightdelay", and we
can alter it (let's say we'll set it to 7 instead of 4), with ";piepokeu
lightdelay 7".
Note that these commands are limited to admins. In host mode, PIE uses the
server admin status to determine who's allowed to run these commands. In local
mode, you will need to specify your account name in the settings.
randomlights.php:
<?php
function PIE_settings()
{
return array('flags' => 0,
'insimname' => 'RandomLights');
}
function PIE_start()
{
$PIE = PIE::connect();
$PIE->set('lightids', array(2,3,4));
$PIE->set('lightdelay', 4);
$PIE->set('lightchanges', 0);
}
function PIE_clock($t)
{
$PIE = PIE::connect();
$delay = $PIE->get('lightdelay');
$delay = max(1, $delay);
if (!($t%$delay))
randomiselights();
}
function randomiselights()
{
$PIE = PIE::connect();
$lights = $PIE->get('lightids');
foreach($lights as $light)
{
$bulbs = mt_rand(0,15);
PIE_sendpacket(array( 'Type' => ISP_OCO,
'OCOAction' => OCO_LIGHTS_SET,
'Index' => AXO_START_LIGHTS,
'Identifier' => $light,
'Data' => $bulbs));
}
$count = $PIE->get('lightchanges')+1;
$PIE->set('lightchanges', $count);
}
?>
> pie tutorials\randomlights.php host.cfg
// [Debugging in code] ////////////////////////////////////////////////////////
Now, we'll do some more debugging - you can never have enough ways to find
out what's going on! We'll take our previous lights program and modify the
randomiselights function.
We'll build two strings to tell us what's going on as each light is set, one
for console debugging now and another saved in an array for outputting to chat
later.
We use the 'PIE_debugout' function to optionally output debugging messages
to the PIE console. The first parameter is the level of debugging that should
be required for this message to be output, the second is the message itself.
Alternatively, we can use the 'PIE_debugcheck' function to see if a certain
level of debugging is enabled, in order to deal with the debugging ourselves.
Here we'll send it to chat. We'll use a different debugging level so that we
can toggle these two debugging options independently.
We also have a new helper function 'textbulbs', to more easily see which
bulbs should be set for comparison. (Note, this is correct only for startlight
objects, main lights follow a different pattern.)
randomlightsdebug.php:
<?php
function PIE_settings()
{
return array('flags' => 0,
'insimname' => 'RandomLights');
}
function PIE_start()
{
$PIE = PIE::connect();
$PIE->set('lightids', array(2,3,4));
$PIE->set('lightdelay', 4);
$PIE->set('lightchanges', 0);
}
function PIE_clock($t)
{
$PIE = PIE::connect();
$delay = $PIE->get('lightdelay');
$delay = max(1, $delay);
if (!($t%$delay))
randomiselights();
}
function randomiselights()
{
$PIE = PIE::connect();
$lights = $PIE->get('lightids');
$debug = array();
foreach($lights as $light)
{
$bulbs = mt_rand(0,15);
PIE_sendpacket(array( 'Type' => ISP_OCO,
'OCOAction' => OCO_LIGHTS_SET,
'Index' => AXO_START_LIGHTS,
'Identifier' => $light,
'Data' => $bulbs));
$debug[] = '#'.$light.': '.textbulbs($bulbs);
$msg = $t.' lights #'.$light.' set to '.$bulbs.' ('.textbulbs($bulbs).')';
PIE_debugout(PIE_DEBUGUSER1, $msg);
}
$count = $PIE->get('lightchanges')+1;
$PIE->set('lightchanges', $count);
if (PIE_debugcheck(PIE_DEBUGUSER2))
{
$msg = 'change '.$count.': '.implode(', ', $debug);
PIE_say($msg);
}
}
function textbulbs($bulbs)
{
$return = '';
$return .= ($bulbs & 1) ? 'R' : '-';
$return .= ($bulbs & 2) ? 'Y' : '-';
$return .= ($bulbs & 8) ? 'G' : '-';
return $return;
}
?>
> pie tutorials\randomlightsdebug.php host.cfg