// [Race start message] ///////////////////////////////////////////////////////
Now let's try sending packets. We'll use buttons instead of chat to send
messages at the start of each race, using the BTN packet.
To send a packet with PIE, we need only make an associative array with the
required fields defined and pass it to the 'PIE_sendpacket' function. PIE sets
default values for all the fields, so you can leave out anything you don't
need. PIE also handles the 'ReqI' and 'Size' fields, so you can forget those
too.
We'll hook the RST packet and send our button packets from there. As we're
making a host InSim, we'll need to specify a UCID to send the buttons to.
We'll use the use the special value 255 to send them to all connections.
Buttons will persist until cleared by another packet, or the user, or the
InSim is closed. So, we'll have to clean it up ourselves after a delay. We
can't wait with a sleep function because PIE needs to keep doing other things
in the meantime, so we will use a callback.
In this case we'll make a 'killbutton' function to send another packet, BFN.
We'll use the 'PIE_setcallback' function to tell PIE when to call our
function, and what value to pass to it as a parameter. (We'd need to use an
array for passing multiple values, but in this case we only need the button's
ClickID). We'll set a time 6 seconds in the future in this case, and pass the
relevent ClickID in the args parameter.
racestartmessage.php:
host.cfg: (set your own host/port/adminpass)
Command line:
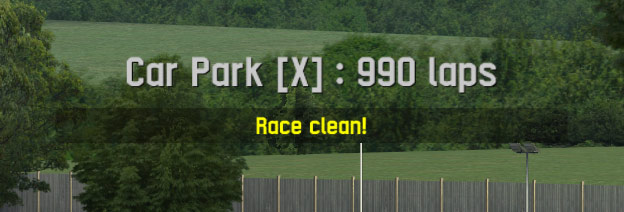
Now let's try sending packets. We'll use buttons instead of chat to send
messages at the start of each race, using the BTN packet.
To send a packet with PIE, we need only make an associative array with the
required fields defined and pass it to the 'PIE_sendpacket' function. PIE sets
default values for all the fields, so you can leave out anything you don't
need. PIE also handles the 'ReqI' and 'Size' fields, so you can forget those
too.
We'll hook the RST packet and send our button packets from there. As we're
making a host InSim, we'll need to specify a UCID to send the buttons to.
We'll use the use the special value 255 to send them to all connections.
Buttons will persist until cleared by another packet, or the user, or the
InSim is closed. So, we'll have to clean it up ourselves after a delay. We
can't wait with a sleep function because PIE needs to keep doing other things
in the meantime, so we will use a callback.
In this case we'll make a 'killbutton' function to send another packet, BFN.
We'll use the 'PIE_setcallback' function to tell PIE when to call our
function, and what value to pass to it as a parameter. (We'd need to use an
array for passing multiple values, but in this case we only need the button's
ClickID). We'll set a time 6 seconds in the future in this case, and pass the
relevent ClickID in the args parameter.
racestartmessage.php:
<?php
function PIE_RST($data)
{
$packet = array('Type' => ISP_BTN,
'UCID' => 255,
'ClickID' => 7,
'BStyle' => ISB_DARK,
'T' => 55,
'L' => 60,
'W' => 80,
'H' => 10,
'Text' => '^3Race clean!');
PIE_sendpacket($packet);
$time = microtime(true) + 6;
PIE_setcallback($time, 'killbutton', $packet['ClickID']);
}
function killbutton($id)
{
PIE_sendpacket(array( 'Type' => ISP_BFN,
'SubType' => BFN_DEL_BTN,
'UCID' => 255,
'ClickID' => $id));
}
?>
flags=0
host=1.2.3.4
port=54321
adminpass=secretpassword
Command line:
> pie tutorials\racestartmessage.php host.cfg